This article will provide you with a step by step guide on how you can build a simple sports odds platform using Cloudbet Feeds API and the new Cloudbet Market Helper package.
The Cloudbet Feeds API provides you with the Cloudbet sports odds required for betting markets and game lines comparison. However the Cloudbet API is best used in conjunction with the Cloudbet Market Helper to help parse all the markets and lines. For a detailed introduction on how to use the Cloudbet API, you can read this article.
The Cloudbet Market Helper is an SDK for the Cloudbet Feeds API published on NPM. By using the SDK, you can read the betting markets and lines and build sports odds comparison platforms free of charge. This is especially useful for Cloudbet Affiliates to conveniently visualise odds comparisons for major sports events from different operators on site to highlight Cloudbet’s favorable odds offers to their visitors and build an odds comparison.
Contents
- 1 Overview of building a sports odds platform
- 2 Getting started
- 3 Displaying markets and events
- 4 Displaying game lines and odds
- 5 Available markets and sports
Overview of building a sports odds platform
The article is split into two main parts. The first part focuses on how to request markets and events data and the second part explains how to display game lines and odds using Cloudbet Market Helper. For the purpose of providing you with step by step instructions in this article, we have chosen React JS to build the UI, however you can use any other javascript framework of your choice. The final product will look like below:
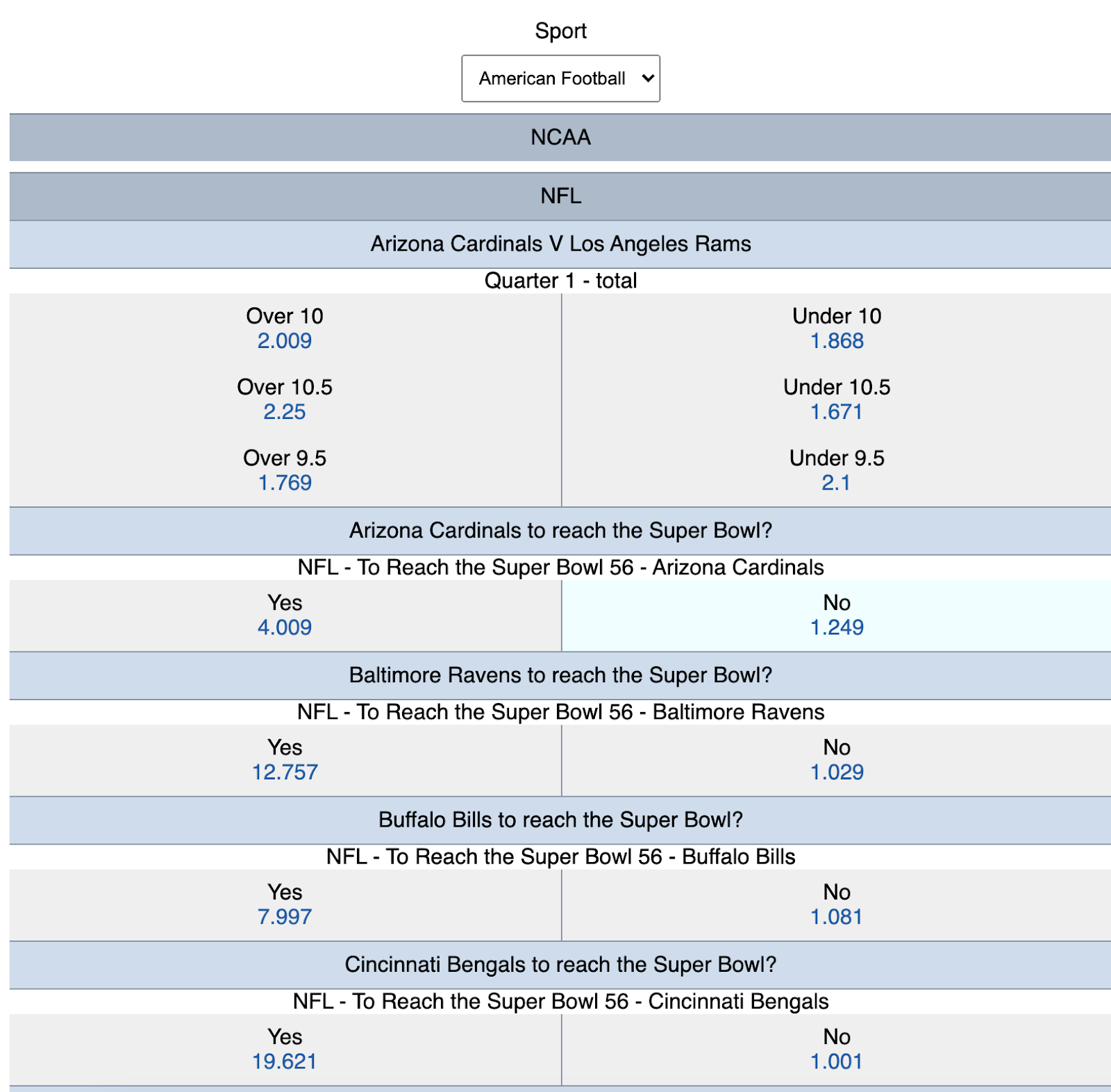
Getting started
Let’s bootstrap from React JS using following commands
or
To test the bootstrap run yarn start
Afterwards you will be able to access the page in the browser and see the below screen.
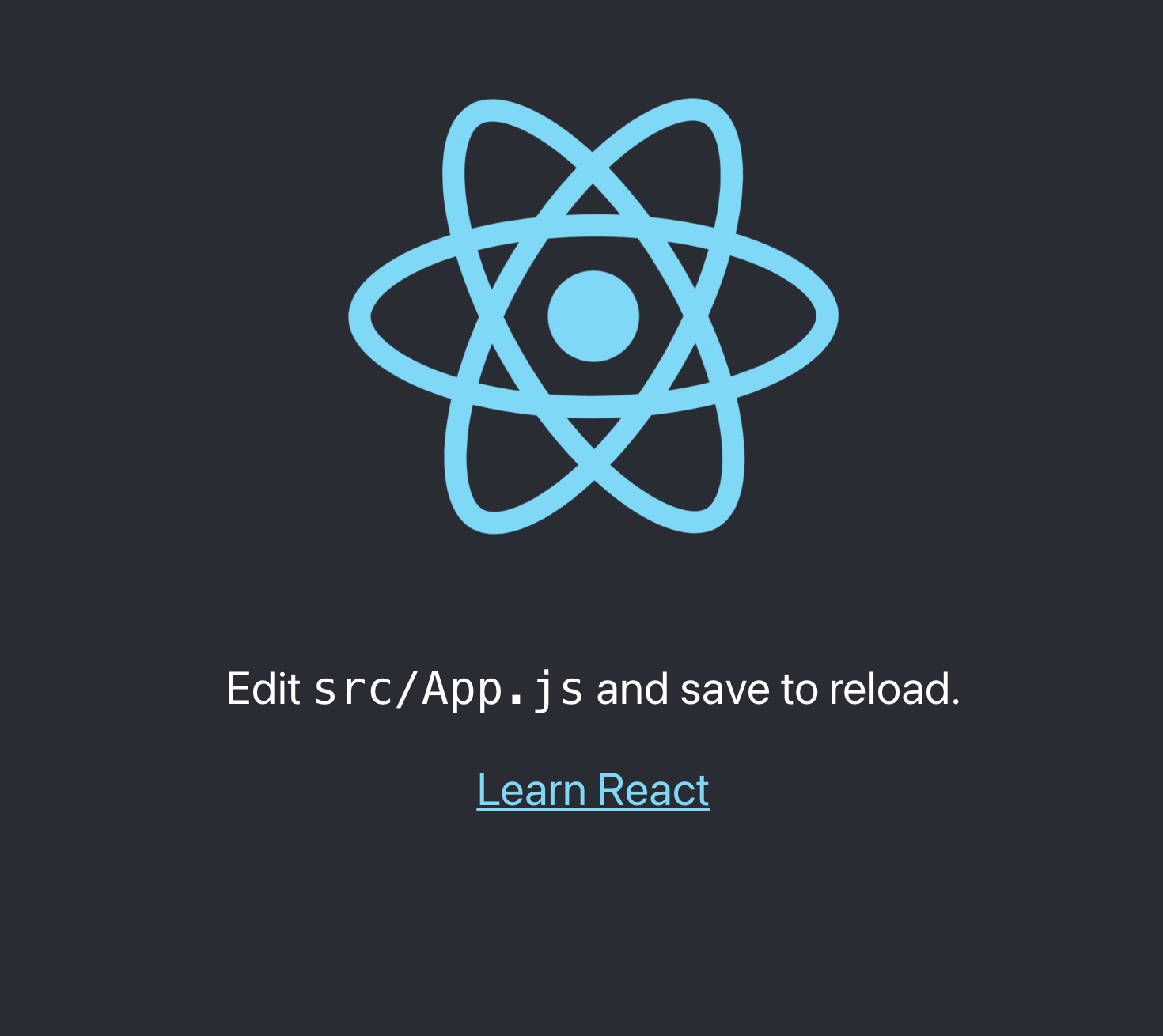
Now we are ready to implement the odds platform on top of this.
Displaying markets and events
Before we start with the retrieval and display of odds, it’s important to understand how our data is structured.
Concepts
- Competition – tournaments, such as NBA
- Event – Contains markets about teams competing. Ex: Cleveland Cavaliers V Chicago Bulls.
- Market – Contains a list of lines and selections to bet on for a particular event. Ex: Winner market.
Specifying sports
In order to retrieve oddsretrieveodds, we need to specify a sport by using a sport key. You can find a list of sport keys here. Let’s use Soccer, Basketball and American Football keys for this app and load them into a select dropdown.
Now you can see the sports in your app as follows.
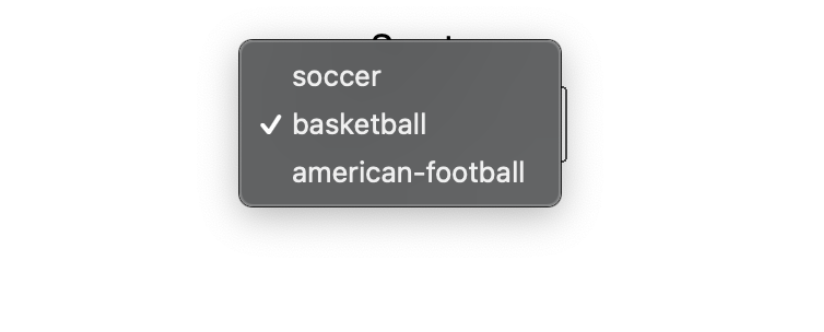
We need to convert them to sport names. Especially when you want to localise your app for a specific language(s), That’s where the market helper comes into play.
Install Market Helper
First you need to install a market helper SDK in your project.
or
Using Market Helper for sport names
You can use the market-helper package to retrieve proper sport names using the sport key and by passing the locale of your choice. For this app, we will be using en. But you can see what locales are available using the Locale type. Also note that the market-helper package will fallback to en if translations are not available. Let’s use the getSportsName method from the market helper and load the proper names to a select dropdown.
Now you can see sport names instead of sport keys in the drop down.
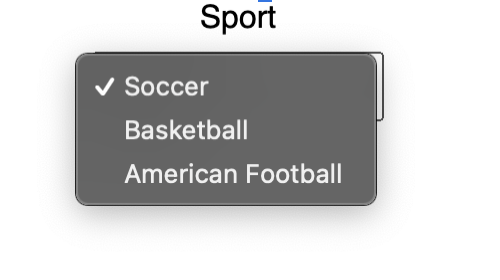
Getting a sport from Cloudbet API
Let’s create a getSport function to fetch sports data using the Cloudbet API.
Now we simply need to obtain an API key as below. There are two types of API Keys: Cloudbet Affiliate API Key and Cloudbet Trading API Key.
Generating Cloudbet Trading API Key
API key for Affiliates
You need an API Key to consume the Cloudbet Feed API and retrieve odds. For that you can use the Affiliate API. It is primarily meant for consuming Cloudbet odds without the ability to place bets programmatically. The retrieved odds may be cached for some time if you opt to use an Affiliate API Key. You can obtain it by signing in as a Cloudbet affiliate and visiting your Affiliates API Token page after signing in.
API key for Trading
Alternatively you can use Cloudbet Trading API Key by logging into your Cloudbet account or by signing up. You can then find your API key in My Account > API section.
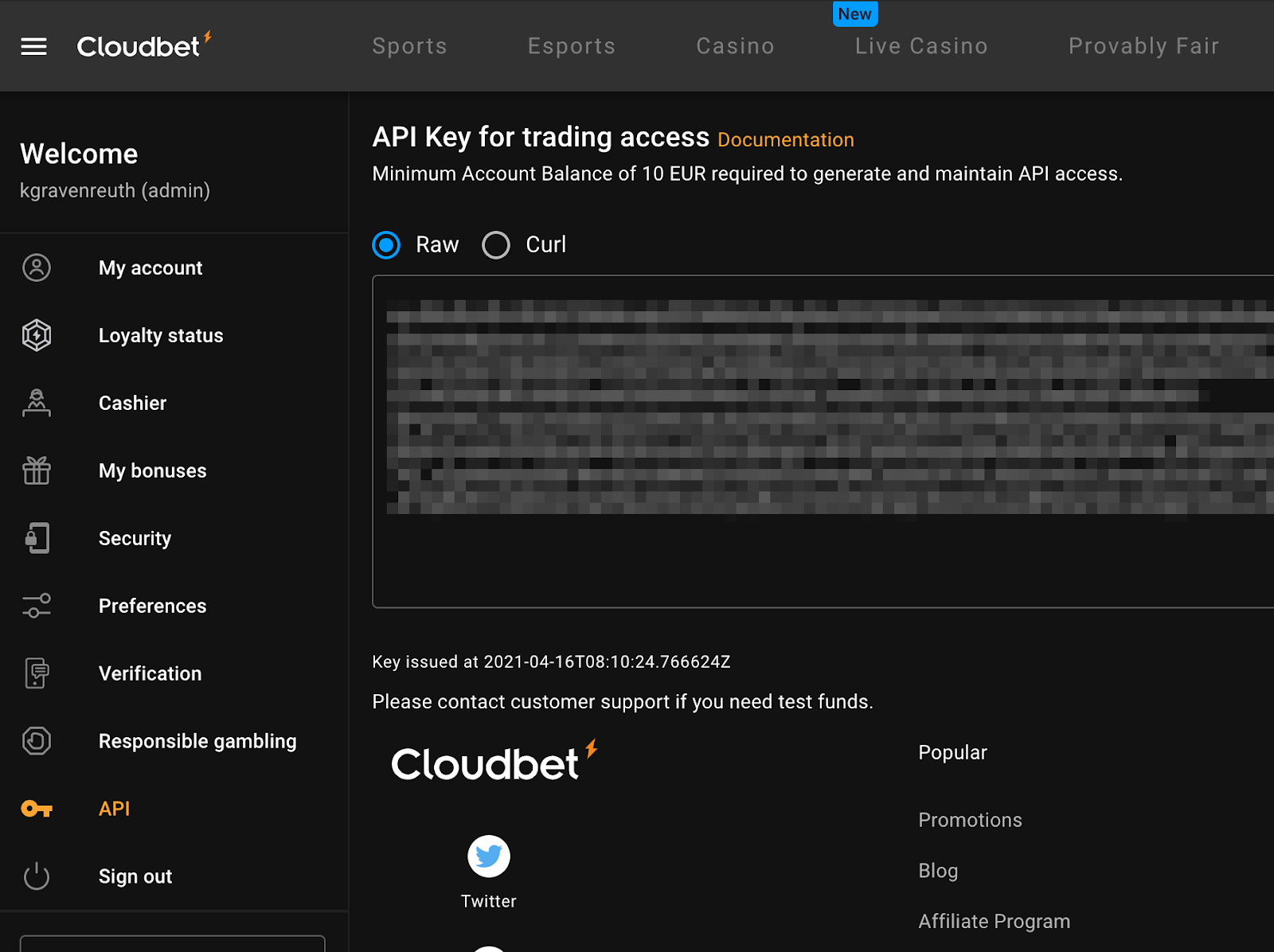
This Cloudbet Trading API Key can be used to fetch sports and competitions data and display them using the market-helper package. In addition, you can use the Cloudbet Trading API Key to place bets programmatically. For placing bets, there are requirements such as minimum balance. You can read more about it here.
You can use this to get the sport data from api by specifying the Affiliate API Key in the X-API-Key request header for the sport endpoint . Since it’s an asynchronous call, we have to introduce a loading state as well, to be shown until the data is fetched. Let’s modify the App.js as follows.
Loading competitions from sports response
Each sport has multiple competitions. In order to display odds, we first need to display the competition. Let’s use the competitions in response and display them in a list.
Now the competitions will be listed as follows.
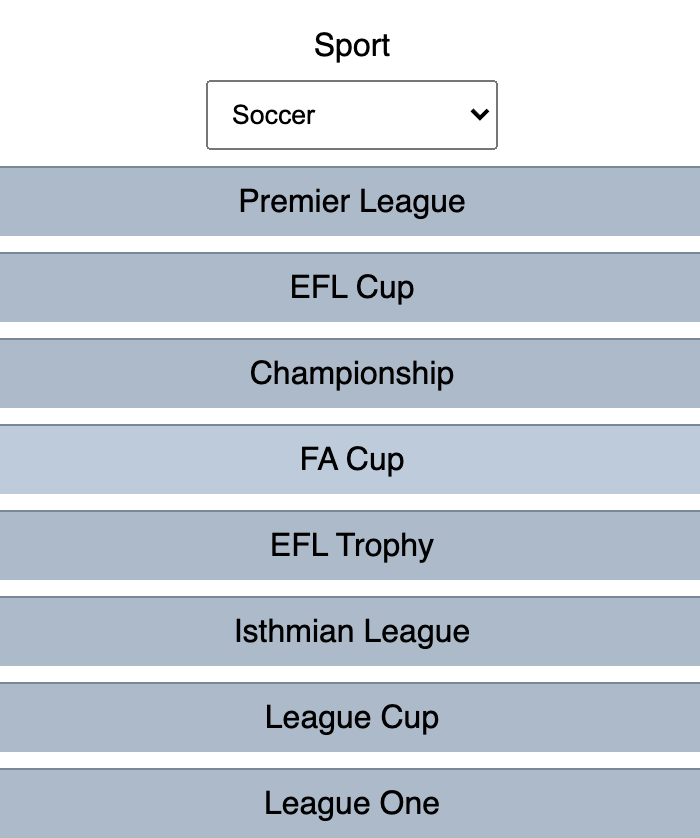
Displaying competition data
Each competition has multiple events. We can use the following function to fetch competition data from the API. Note the competition endpoint used here.
In order to display a competition, let’s create a separate Competition.js component and use the above getCompetition API function to load the events into the competition upon clicking on a competition’s name and display a list of Event IDs as follows.
Now import the Competition and modify the JSX in App.js to display the event under competition as follows.
Now you can see a list of Event IDs upon clicking into a competition.
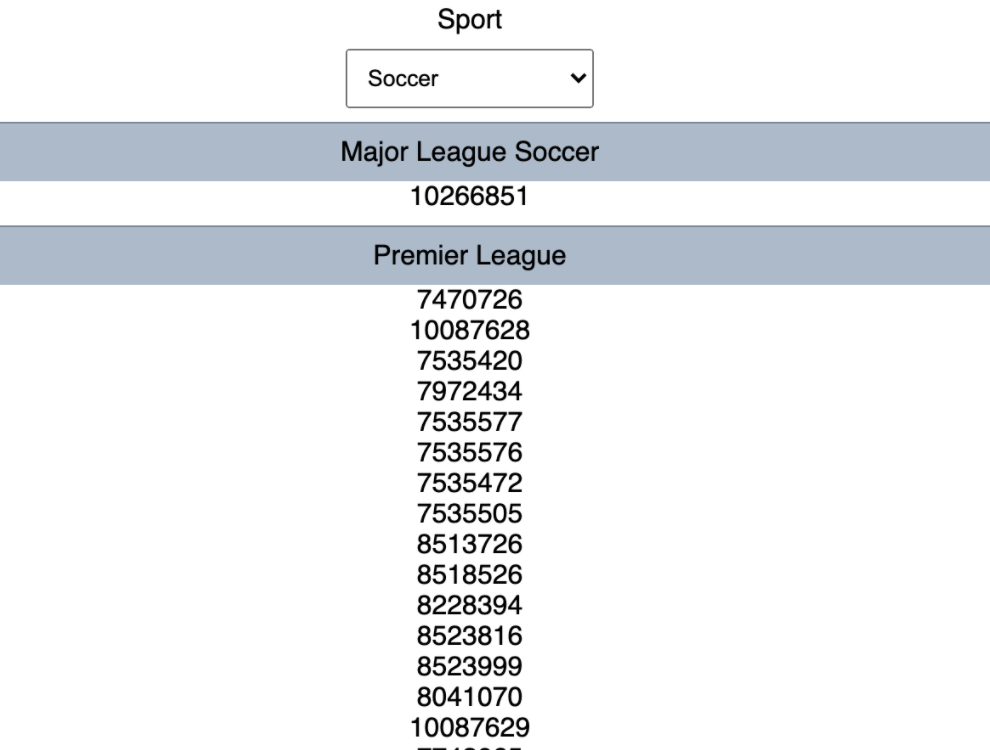
Displaying game lines and odds
Event Data from the API will contain the following items.
- Line – Odds for multiple outcomes of a given market.
- Outcome – Possible result for a market. Ex: Home Team wins, Away Team wins or draws.
- Odds – Out of all outcomes, likelihood of a particular outcome to occur in the game.
Each competition has multiple events that consist of lines for different markets. In order to read and display odds for these events, we need to specify the markets as follows using the MarketType and use getMarket methods from the market-helper package and pass the event to get the market details as follows.
Displaying odds for simple markets
Let’s first start with one simple market from each sport.
A Market has multiple lines that consist of outcomes. Each outcome has variables and back values. Variables will contain either of the following.
Result – Market outcome to be a given competitor winning, losing or draw.
total – Market outcome to be over or under a total value. Ex. Total goals to be over/under 1
handicap – Asian Handicap value for the market. See handicap betting for more details.
Now let’s adopt the above code and create a new Event.js component to load and display the odds for these markets. We will be using a back price for each outcome. Back price is the value for the outcome that you’re backing. Ex. You’re backing a particular team to have a number of goals above given value at the end of first half by betting on it. Market type for that would be soccer_total_goals_period_first_half.
Finally we can use the event component in the previously created Competition component and display the odds by importing the Event component and modifying the JSX as below.
Displaying game line for game final result
Let’s select Soccer from the dropdown and you can see the line for the game result as follows for each event.
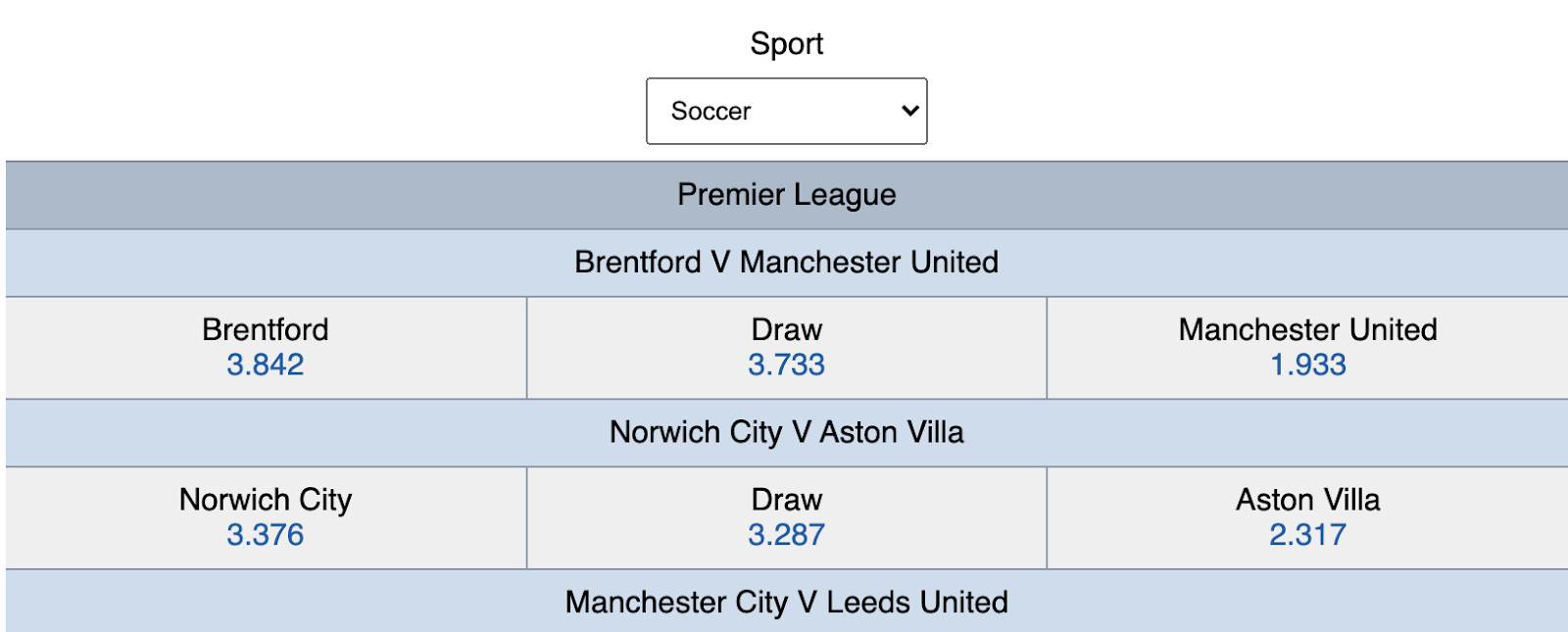
Here, the getMarket function from the market-helper package will populate the outcome name and provide you the odds value so you can get the line and display it.
Displaying line for over/under
Let’s select American Football from the dropdown and you can see the lines for the game result populated into markets returned by getMarket function as follows.
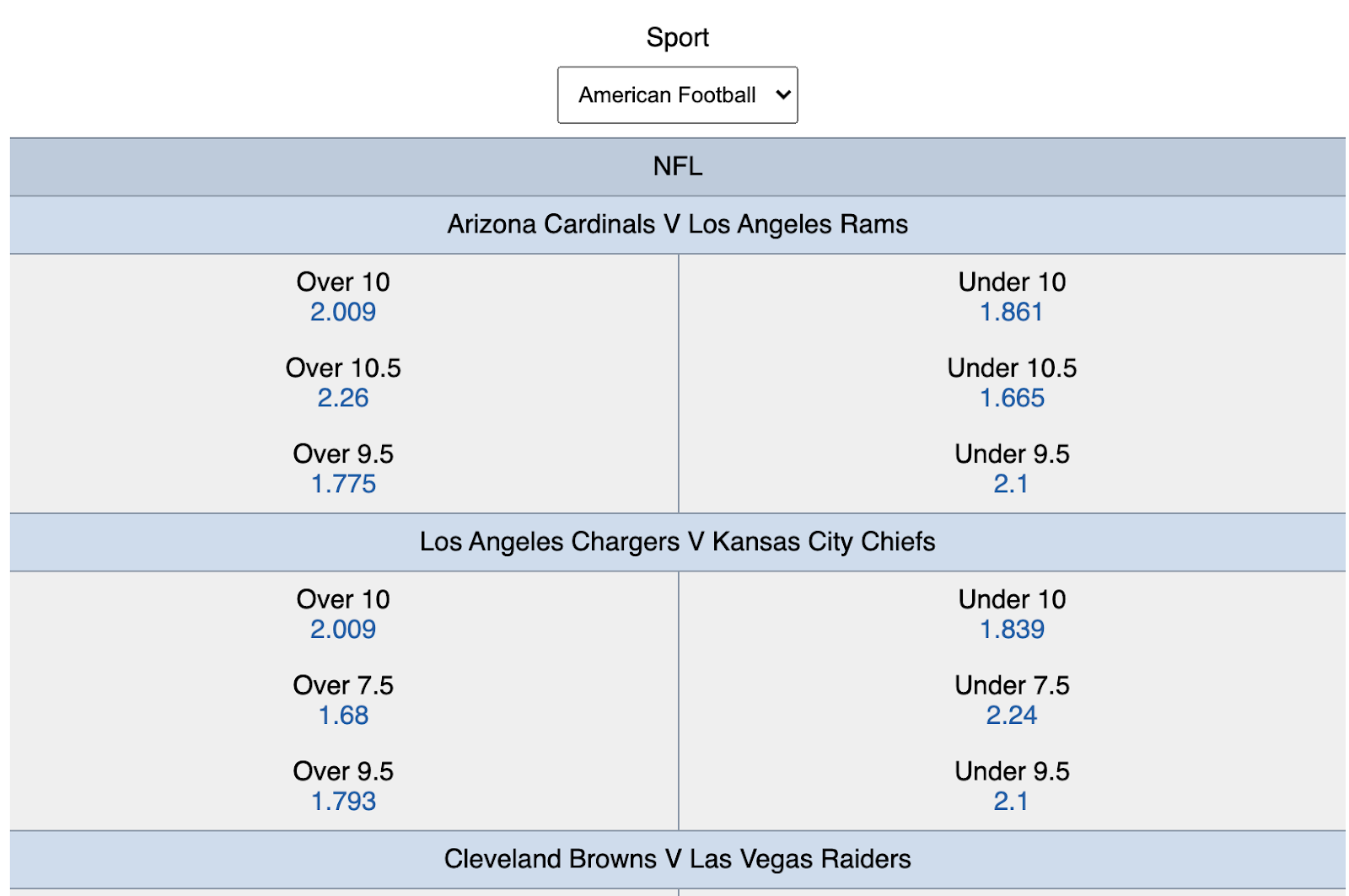
Displaying Handicap lines
Now let’s improve our odds platform by adding more lines. For that, I am going to use the Soccer Asian Handicap market. Let’s assign it to the soccer market config as follows.
Modify the React Memo to load all the lines of all the markets of selected sport.
Now if you select soccer from the dropdown, you will see both final result and handicap game lines displayed together as follows.
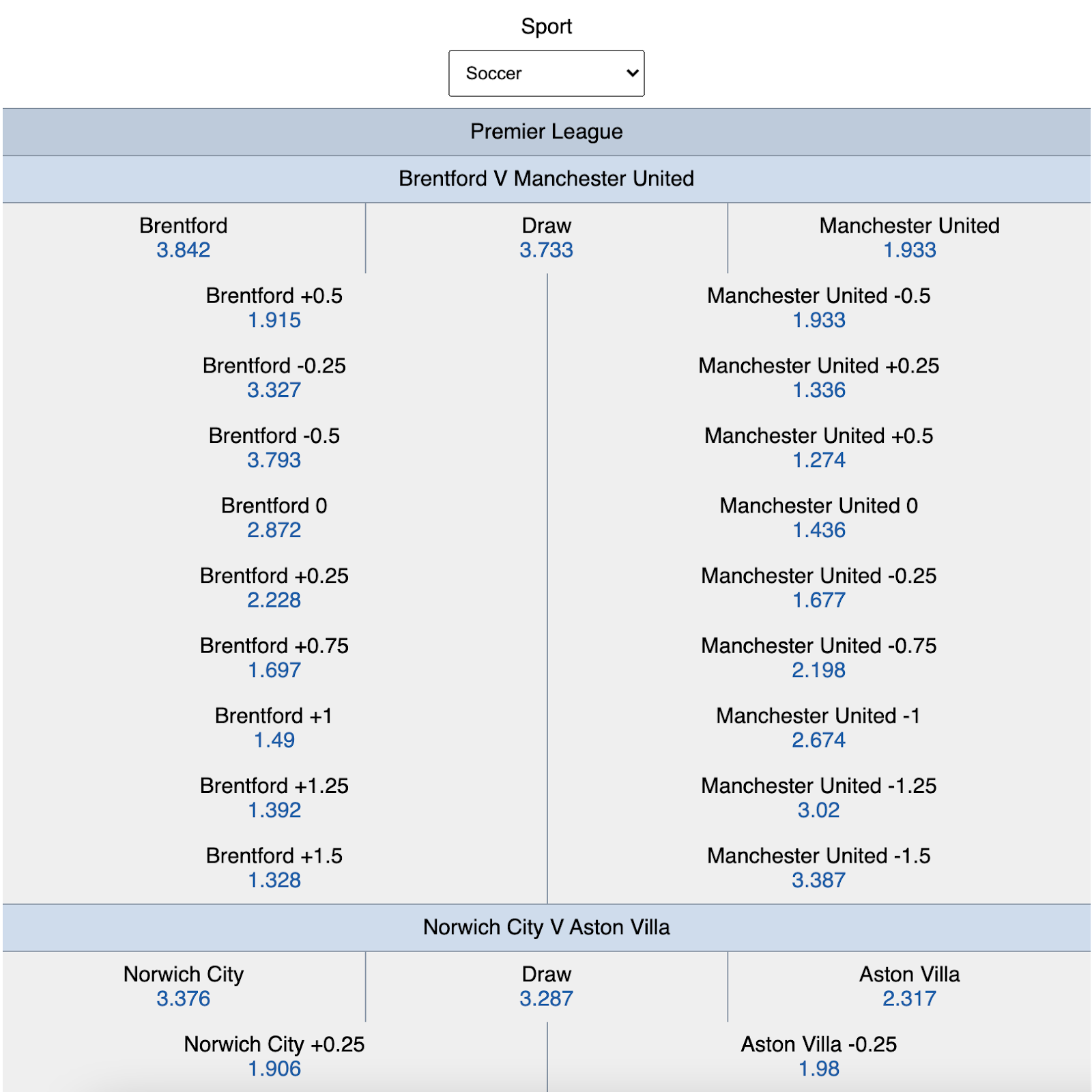
After we specify the handicap market, getMarket populate the handicap value of each outcome into market.name and provide lines based on different handicap values. First entry in the lines array is the primary line. Alternative lines are sorted based on Handicap value in asc order. But this does not apply for ICE Hockey or Baseball where the run line 1.5 is the primary line.
Now let’s use additional data populated in the market from getMarket and display market names above respective lines by modifying JSX in the Event component.
Now you will have lines categorised by market name as below..
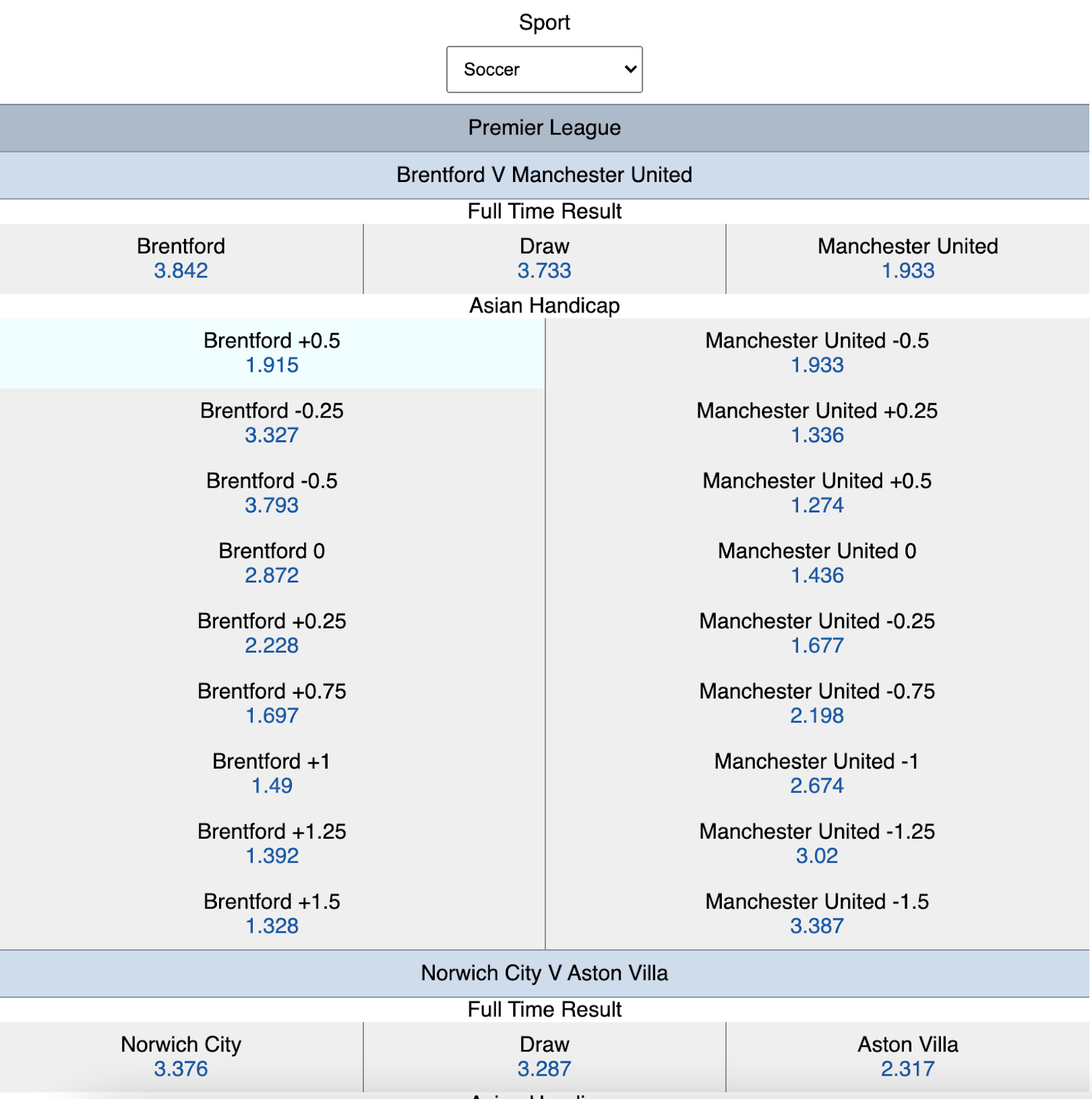
Displaying outrights
We can further modify this to display outright markets. Let’s add american football outrights market to market config.
Now the market-helper package will populate outright markets for the competition with other markets and display them as follows after quarter total lines.
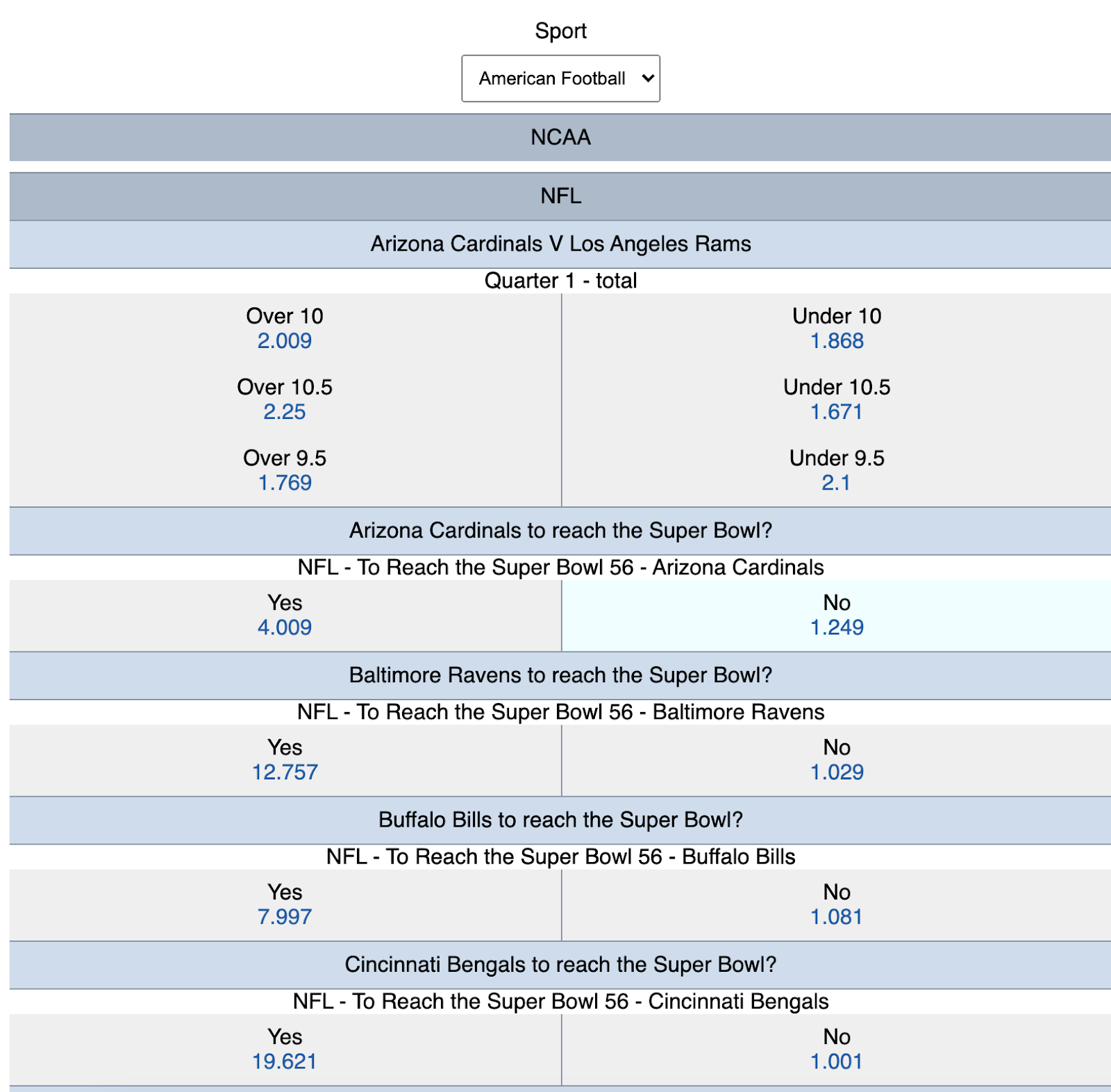
Displaying game lines in one view
For some markets, instead of separating the lines, we can rearrange the outcomes to show the lines in one view. In order to do that, let’s first modify the basketball market config as follows.
Now you will see these additional markets displayed as follows.
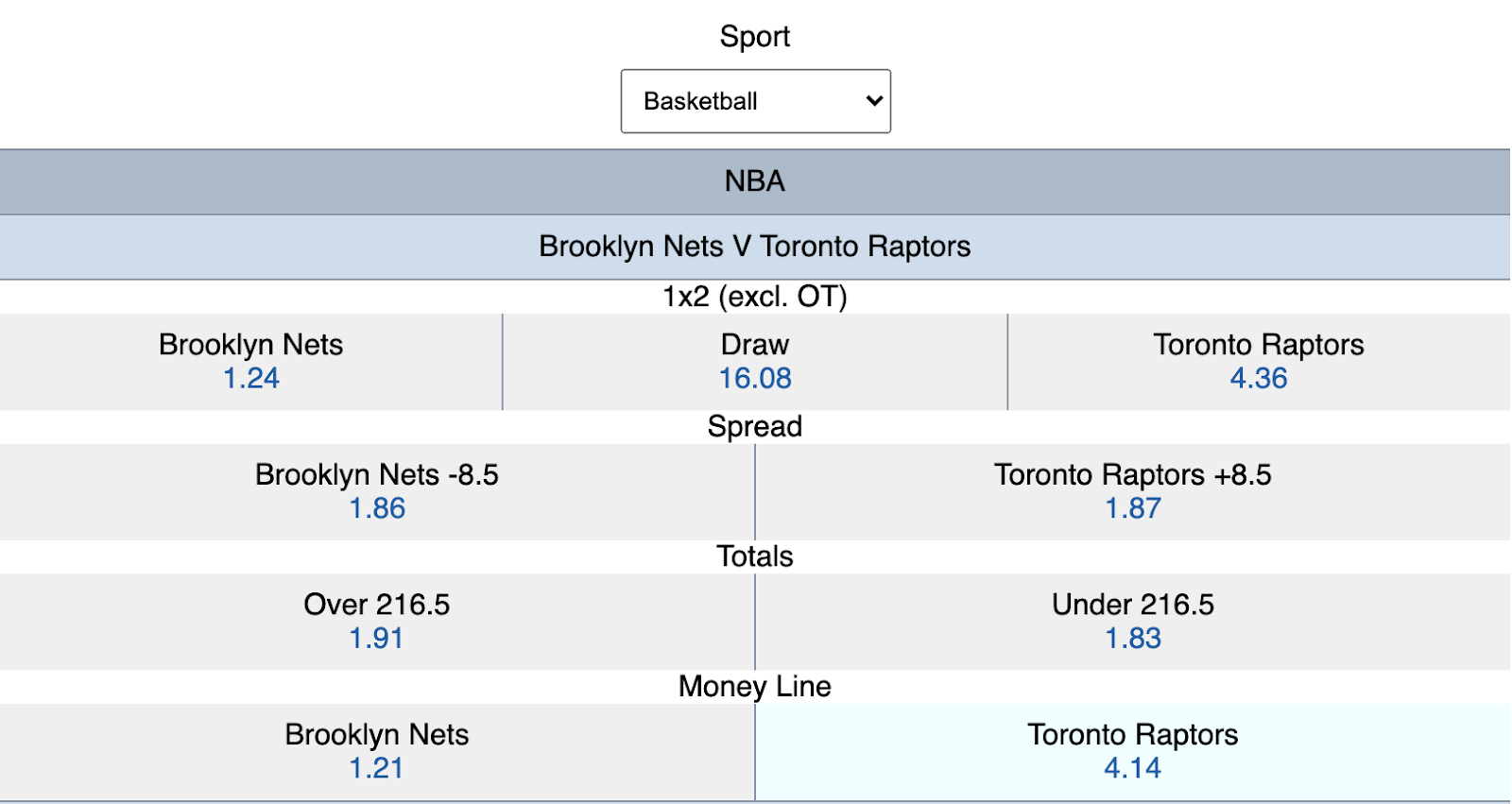
Let’s create a new component to arrange this view as follows to show game lines in one view. First, separate the three markets out as game line markets.
Create a new React Memo to store the odds data needed to render in a new view. We consider the first outcome (array element) in the line as the home team and second one as the away team.
Now let’s combine these and create a GameLines component as follows.
Modify the JSX in the Event component by adding the GameLine component at the bottom.
Now let’s select Basketball and see how the Game Lines view is rendered.
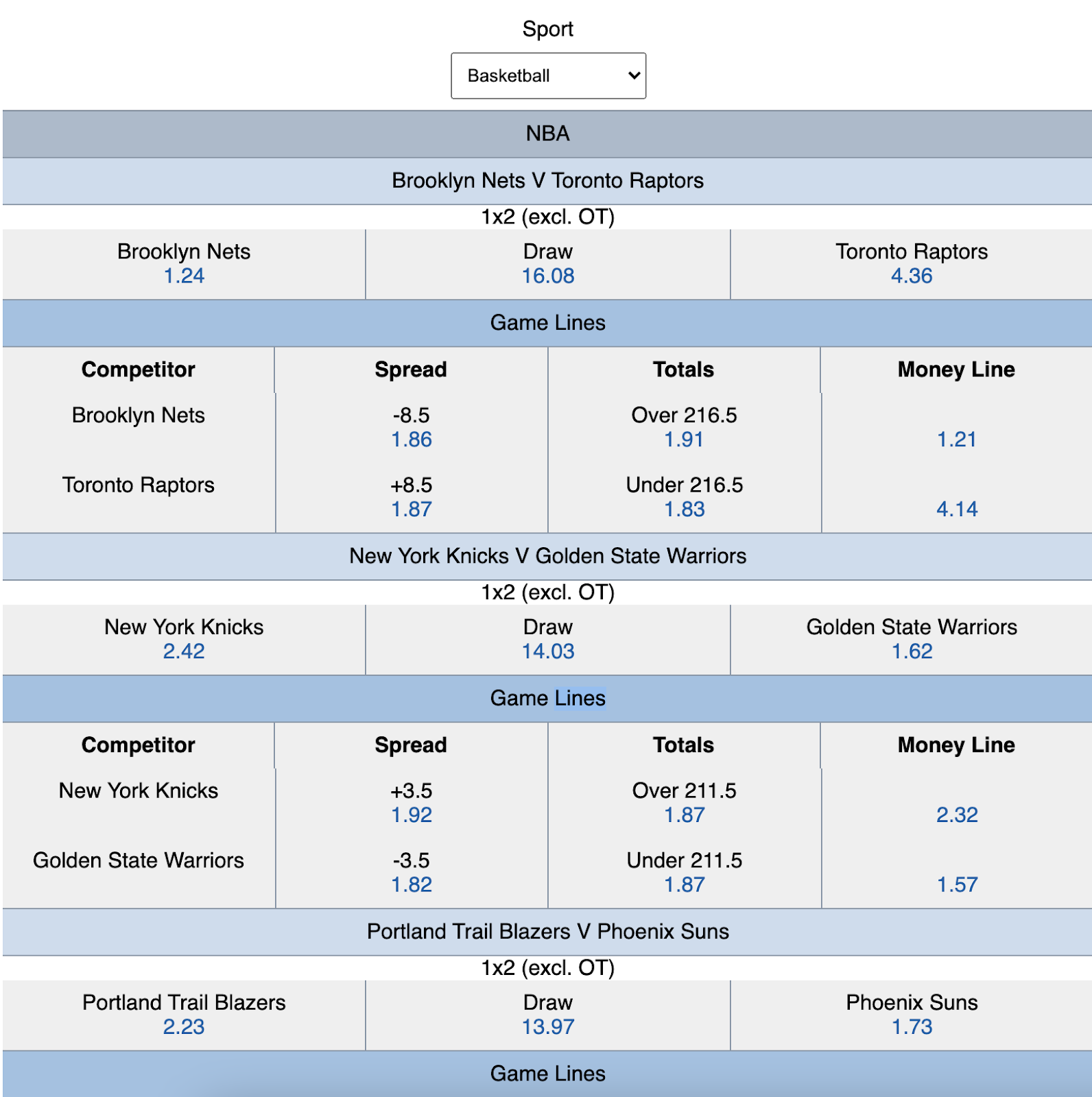
Available markets and sports
For a full list of markets and sports, please see this Github gist.